Chapter 4 Flow of Code
At this point, it is helpful to discuss how Python executes multi-line programs. Here are the rules.
4.1 Rule 1 - Top to Bottom
In general, a Python program runs one line at a time starting from the first sentence and finishing at the last. This flow can only be changed by using special Python keywords.
i=1
j=10
k=i*j+7
print(i,j,k)
The code execution flow for the above program is shown in the following figure.
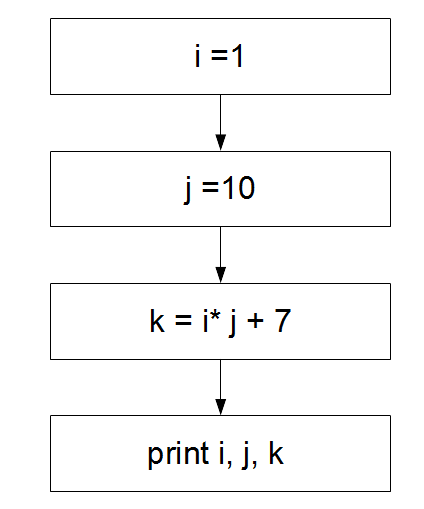
Code Execution Flow
4.2 Rule 2 - Right to Left
When Python encounters an “=” sign in a line, it first computes the right hand side of the expression and then stores the result in the variable on the left hand side. Therefore, you cannot have code like the following.
x+7 = 9
4.3 Rule 3 - Comments
Python ignores empty lines. So, you can sprinkle them in your code to improve readability. Also, if a line contains the character ‘#,’ all text to the right of ‘#’ are ignored.
4.4 Rule 4 - Variables
Python variables are like boxes with name and holding some value inside. At one time, a box can hold only one value.
x = 1
y = 5
x = y + 3
y = x - 3
print (x,y)
In the above code
after the first line runs, Python creates a box named “x” and holds the value 1 in it.
after the second line runs, Python has two boxes, one named “x” with the value 1 in it and the other named “y” with the value 5 in it.
after the third line runs, box “x” gets the value 8 (current value of “y” plus 3, while “y” remains as 5.
after the fourth line runs, box “y” gets the value 5 (current value of “x” minus 3), whereas “x” stays as 8.
after 5th line, the code prints 8 and 5.
4.5 Changing the Standard Flow
In standard flow, a python script starts from the first line in a file and continues running the code in each line until reaching the last line in the file.
There are situations, where you may not want to execute every line once. You may want some line to be run five times with five different parameters. You may also choose to skip lines, if certain condition is not met. Special keywords (for, while, if, def ) are used to break the flow of sequencial execution.
- The ‘for’ block allows running the commands within a set of lines multiple times.
for i in [1,2,3,4,5,6,7,8,9,10]:
print("5 times", i, "is", 5*i)
- The ‘if’ block allows running the commands within a set of lines only if a condition is satisfied.
i=7
if(i<10):
print(i, " is less than 10")
else:
print(i, " is greater than 10")
- The ‘while’ blocks allow running the commands within a set of lines multiple times.
i=1
while(i<=10):
print(5*i)
i=i+1
- The ‘def’ block gives a name to a set of lines of code. Afterward in the code, whenever the programmer wites the name, Python automatically executes those lines of code.
def square(x):
return x*x
print square(2)
print square(3)
We will see examples of all four methods in the following chapters.